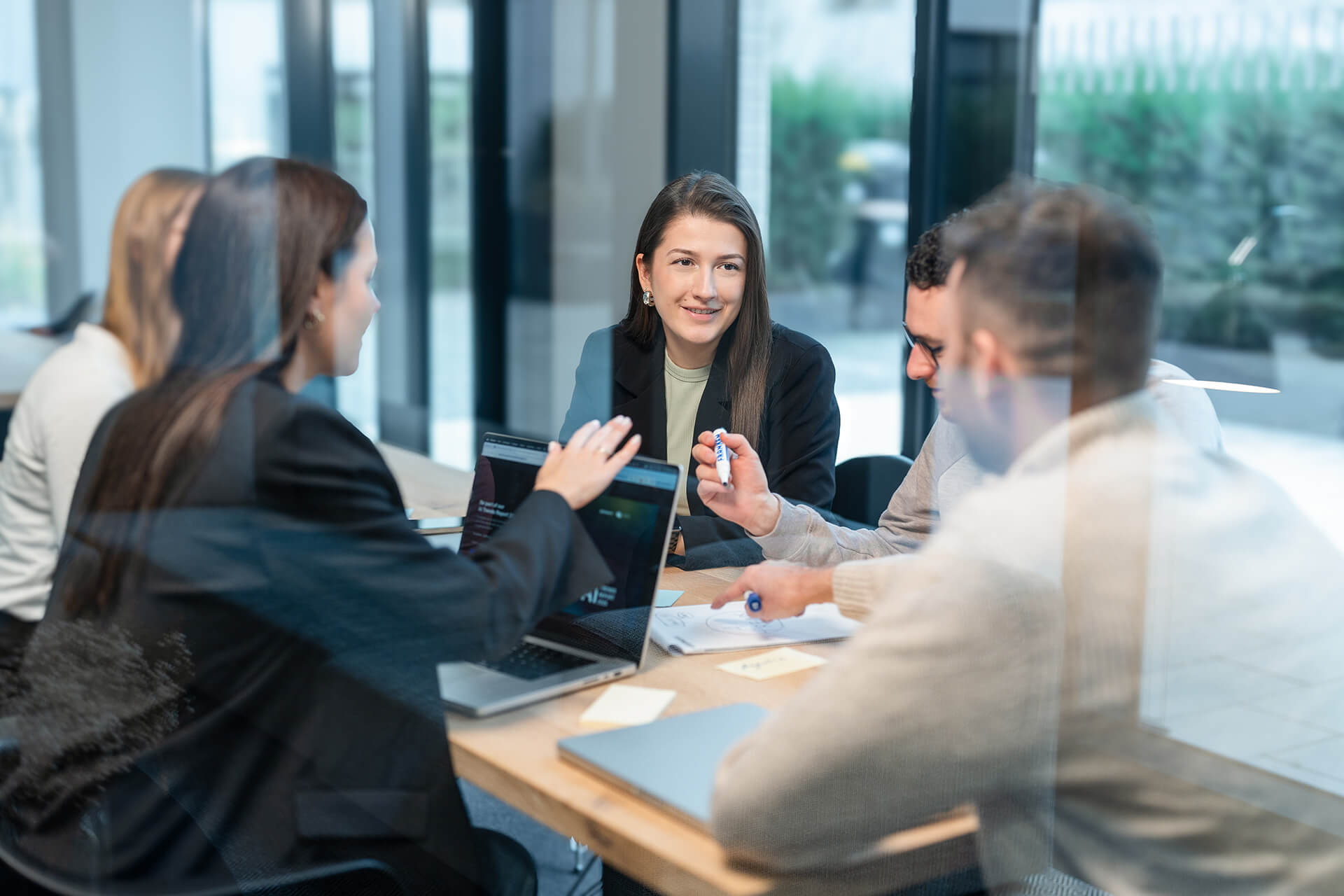

statworx is one of the leading consulting and development companies for Data and AI in the DACH region. We are more than just a service provider – we are your partner for the entire AI transformation. We consult, we develop, we provide further training – for more than 10 years, in over 1.000 Data & AI projects, and for over 100 clients from almost all industries. Our experts understand which tech trends truly enhance your business.